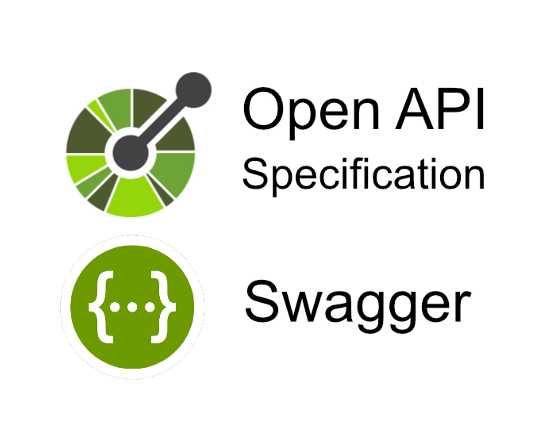
Swagger API Documentation in PHP
Today, we will learn why do we need the API documentation and how to create API documentation.
First lets begin with why do we really need the API documentation, To understand this problem, we will have to think how do we share our APIs with different team after its created/deployed/implemented.
Earlier we used to share the postman collection with different teams or if you are from ASP.net then you might have used WSDL file(pronounced as wis-dal) to share your APIs. But After some point It becomes very difficult to manage these postman collection or WSDL files(and you end up with multiple version of your “API document” shared with multiple teams).
To solve this problem, to centrally manage our APIs documentation so that frontend developers can very easily access our APIs without any worrying about which version of document to refer to, we can use Swagger.
Swagger allows us to create APIs documentation or Specification for our APIs which then can be hosted on single server with other Swagger Tool called Swagger UI, which consumes this APIs Specification file and shows us very beautiful UI for interacting with our backend.
Frontend developers can use this tools to interact with backend without even writing the mock application, which gives them sense of assurity what they can expect.
So in this blog we will see how to implement this in PHP code. Basicallyy we will be using annotation to define our APIs which can be extracted by other swagger tools to generated these specification.(ofcourse you can also write the specification manually either in JSON or YAML file).
To start with we will have to define the details about APIs like name, version, developer contact details, term and condition for using APIs etc. And this is done with following block of comment in your code.
/**
* @OA\Info(
* title="Note API",
* version="1.0.0",
* @OA\Contact(
* email="[email protected]",
* name="Backend Developer Team"
* )
* )
*/
Note: You will have to use block comments style to document you api specification in.
After this you can start defining your APIs with other comment blocks inside your code. Normally we define these details on controllers method(if you are from laravel background) but its nor necessory, you can even created new files just for your api defination like “api-spec.php” inside your source code.
/**
* @OA\Get(
* path="/hello-world",
* summary="Return hello world",
* tags={"Hello"},
* @OA\Response(
* response=200,
* description="hello world",
* @OA\Property(type="string")
* )
* )
*/
function helloWorld()
{
$response = [
"code" => 200,
"message" => "All routes",
"content" => $routes,
"request_at" => time()
];
return response("hello World!");
}
@OA\Get
allows you to defines HTTP verbs used to call this API endpoint. Its also takes some config details to defined this endpopint like whats the endpoint of API, whats the request body, what kind of response this API will returns. Additionally we can also include some example.
tags config allows us to group these APIs into multiple blocks. for eg in case of e-commerce we can define all of our product api under “Product” tags while orders API can be defined under “Orders” tag.
There are other verbs also available like Post, Delete, Patch , Put etc.
@OA\Response
Allows use to define the response that this API will return. Api can return different type of response, so we can use multiple @OA\Response to define each response body for example we can have 200 status code when product found in backend and 404 for products we are not available in our backend. We can define two different response body in such cases. Response can be of type JSON, XML etc.
@OA\Parameter
Allows us to define input parameters to our APIs. These parameter can come from query string, request path or header. You can also mark these parameter as required/optional.
@OA\RequestBody
Allowes us to defined the request body for our api endpoint. It can be JSON, XML, binary or any other format that you wish.
Note: By default request/response content type is json. You can modify the content with
@OA\MediaType
.
For more complicated request/response, you case use @OA\Schema
to define models which then you cam also reuse across your APIs documentation.
There are many more tags available which allows you to defined much more complicated apis very easily.
Read more about Swagger documentation at official website : Swagger API
PHP Tools to generate documentation – Swagger PHP
Thanks for reading…