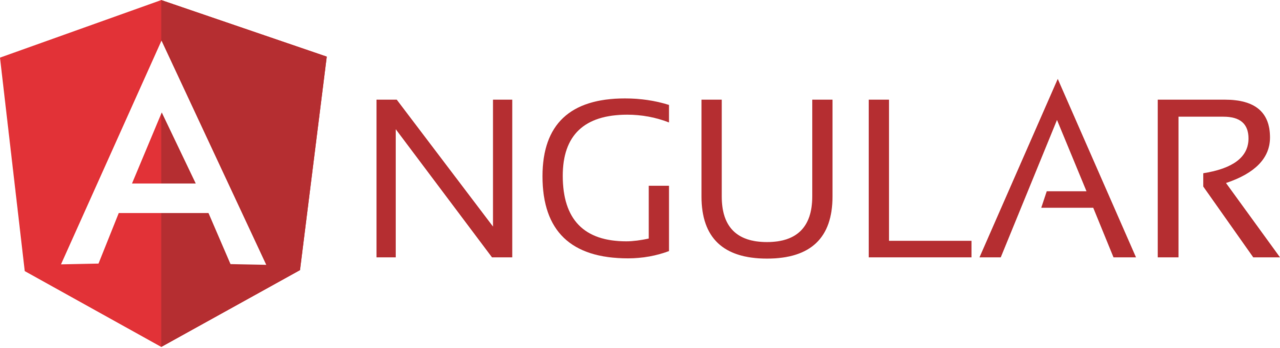
Angular – adding auth token
Hello everyone, In this blog I will show some of the ways you can add tokens or more specifically authentication token e.g., JWT, API Token etc in your API request.
As you most of dev’s already know it, to interact with HTTP web service, Angular provides build in library called HTTPClient, So in this blog we will explore this module, but you could apply this technique to any other library as well.
Ways to add Authentication Token
- Manually in each request
- Using HTTP Interceptor
Manually in each request
In this approach, You manually modify the request i.e., add tokens to each request manually.
let token = this.getApiToken();
const httpOptions = {
headers: new HttpHeaders({ 'Content-Type': 'application/json', 'Accept': 'application/json' })
};
if (token) {
httpOptions.headers = httpOptions.headers.append('token', token);
}
return this.http.get(this.getAPIUrl('note/' + slug), httpOptions)
This is very naive approach if you have multiple API endpoints and also error prone and tedious to maintain it at each API endpoint.
One benefit of using this approach is you get more direct control over the auth token.
Using HTTP Interceptor
In this approach, You create an interceptor which basically acts as middleware, which allows you make any modifications in your request or response before request is sent to server or response received by your application.
You could use interceptor to show global loader as well in your application or add some logging in case server error occurs like showing something went wrong with requestId so that it’s easier to debug the issue.
Following examples show’s how you can create interceptor and attach it your modules.
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>,
next: HttpHandler): Observable<HttpEvent<any>> {
const token = this.getApiToken();
if (idToken) {
const cloned = req.clone({
headers: req.headers.set("Authorization",
"Bearer " + idToken)
});
return next.handle(cloned);
}
else {
return next.handle(req);
}
}
}
In your main module, add following in providers array. This register’s our new interceptor in that specific module.
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true }
This also gives you contol of managing your token at single places, i.e., if in future you want to update your token form bearer to something else, you can just update your interceptor and it will be reflected in all API calls.
if you are using multiple Webservices which are getting served with different domain, you can use this interceptor to only send your auth token to relevant webservice or send different token to each webservices.
Thanks for reading…